Dependency Injection
We have seen that we can utilize the Apex Type class to give us some kind of “dynamic processing”, well, not quite yet. It’s true that we can simplify things now but there are a couple of components in our structure that are still missing.
So far we have one interface and two classes that are implementing our interface. Now we are going to add the dynamic part that makes dependency injection so powerful. All we want is to supply our code with the correct class based on the user that is executing the apex transaction.
We want this to be dynamic and we want one line of code to make that decision for us. Should I go ‘right‘, should I go ‘left‘… we need to control de ‘cross-road’ and point the user on the right direction.
We established that user one needs to handle car orders and that user two will do the same for trucks. We have two service classes, one for each process. Now we need to make sure that these users are sent to the right class to make sure they are working on the expected business process.
For this operation to be successful, and for this particular scenario, we need Hierarchical Custom Setting.
Go to your org and click on Setup -> Custom Code -> Custom Settings.
Click on New
Label: VehicleOrderSettings
Object Name: VehicleOrderSettings
Setting Type: Hierarchy
Let the rest as it is.
Click Save
After this is done you should have your custom setting ready:
![]() |
Inside the new custom setting there is a section Custom Fields, let’s create one field. From that section:
Click New
Select Text from the Data Types
Click Next
Field Label: VehicleOrderService
Length: 80
Field Name is auto-populated
Click Next
Click Save
Great! we are getting closer..
Back to the Custom Setting VehicleOrderSettings, we can see now that there is a new custom field.
Let’s configure our new field.
Click on the button Manage from the top.
After that, we want to click on the bottom New option here.
Location: User
Search for the User that is going to take care of Car orders by clicking on the magnify glass.
VehicleOrderService: CarOderService
Click Save
The user assigned here is going to use the CarOrderService class as you probably know already. Here is where we establish what “road” to take from the “cross-road”. The logic we are using is just the current user which is part of this transaction.
Repeat this operation for user two. Make sure that you use a different user and use TruckOrderService on VehicleOrderService instead of CarOderService.
After that is completed we have practically built all we need, umm… almost.
We need to write a small class that is going to take the role of a factory class.
[code lang=”php”]
public with sharing class VehicleOrderFactory {
// We are instantiating here the hierarchy custom setting and
// getting back the class we need based on the current user
public static IVehicleOrder getVehicleOrder () {
return (IVehicleOrder)Type.forName(VehicleOrderSettings__c.getInstance().VehicleOrderService__c).newInstance();
}
}
[/code]
As you can see, it is a very simple class that contains the Type class operation that will select the class name based on the values which are part of VehicleOrderService__c field in our custom setting. We have configured this field to give us an instance of two different classes TruckOrderService and CarOderService. And we decided that each class should be assigned to two different users.
All you need to do, to test if it works, is to log in with User one and execute an anonymous window from you developer console. On the that execute anonymous we are going to call the factory class like this:
[code lang=”php”]
IVehicleOrder vehicle = VehicleOrderFactory.getVehicleOrder();
vehicle.engine(); vehicle.gps(); vehicle.color();
[/code]
The out put should be something similar to this:
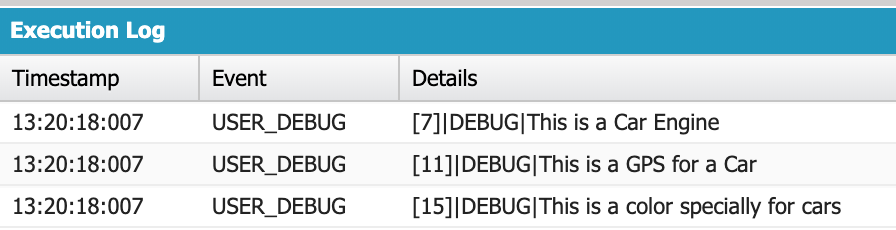
If you log out with User one and log back in with User two, after running the same code on a execute anonymous window you should get something like this:
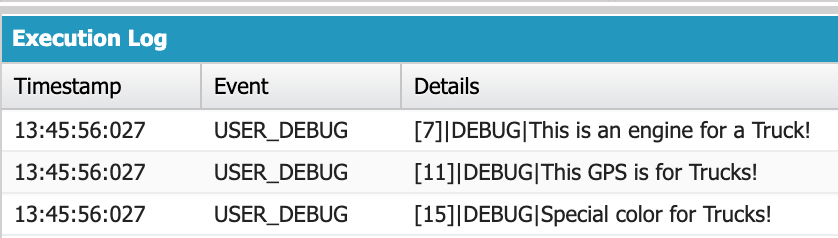
This is pretty much what dependency injection is all about. This is a very simple example that is probably not the best scenario but it helps to understand the basic concepts of this technique. I hope this helps to clear out doubts about this subject. Happy coding!