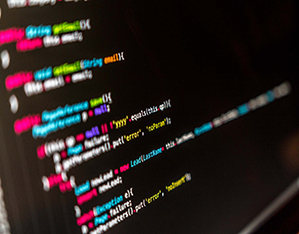
Access SObjects names dynamically from a List
Access SObjects names dynamically from a List A simple way to do this is by using getSObjectType().getDescribe().getName(); You can run that piece of code in your developer console. [code lang="php"] /********************************************************************** * * Access SObjects names dynamically from a List ************************************************************************/ List<SObject> objects = new List<SObject>(); Account account = new Account(Name = 'AccountSObject'); Contact contact = new Contact(LastName = 'ContactSObject'); objects.add(account); objects.add(contact); System.debug(objects.size()); for(SObject sObjectNames : objects) { String sObjName = sObjectNames.getSObjectType().getDescribe().getName(); System.debug(sObjName); } /* * */ [/code]